Call by Value and Call by Reference
In C programming
language, variables can be referred differently depending on the context. For example, if you are writing a program for a low memory system, you may want to avoid copying larger sized types such as structs and arrays when passing them to functions. On the other hand, with data types like integers, there is no point in passing by reference when a pointer to an integer is the same size in memory as an integer itself.

Now, let us learn how variables can be passed in a C program.
Pass By Value
Passing a variable by value makes a copy of the variable before passing it onto a function. This means that if you try to modify the value inside a function, it will only have the modified value inside that function. One the function returns, the variable you passed it will have the same value it had before you passed it into the function.
Pass By Reference
There are two instances where a variable is passed by reference:
- When you modify the value of the passed variable locally and also the value of the variable in the calling function as well.
- To avoid making a copy of the variable for efficiency reasons.
Let's have a quick example that will illustrate both concepts.
xytotals.c:
Sample Code
- #include <stdio.h>
- #include <stdlib.h>
- void printtotal(int total);
- void addxy(int x, int y, int total);
- void subxy(int x, int y, int *total);
- void main() {
- int x, y, total;
- x = 10;
- y = 5;
- total = 0;
- printtotal(total);
- addxy(x, y, total);
- printtotal(total);
- subxy(x, y, &total);
- printtotal(total);
- }
- void printtotal(int total) {
- printf("Total in Main: %dn", total);
- }
- void addxy(int x, int y, int total) {
- total = x + y;
- printf("Total from inside addxy: %dn", total);
- }
- void subxy(int x, int y, int *total) {
- *total = x - y;
- printf("Total from inside subxy: %dn", *total);
- }
Here is the output:
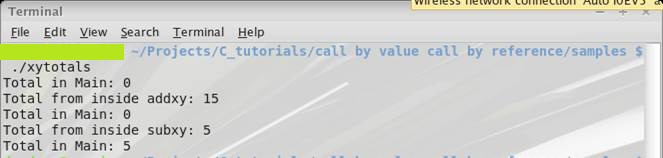
There are three functions in the above program. In the first two functions variable is passed by value, but in the third function, the variable `total` is passed to it by reference. This is identified by the “*” operator in its declaration.
The program prints the value of variable `total` from the main function before any operations have been performed. It has 0 in the output as expected.
Then we pass the variables by value the 2nd function addxy, it receives a copy of `x`, `y`, and `total`. The value of `total` from inside that function after adding x and y together is 15.
Notice that once addxy has exited and we print `total` again its value remains 0 even though we passed it from the main function. This is because when a variable is passed by value, the function works on a copy of that value. They were two different `total` variables in memory simultaneously. The one we set to 15 in the addxy function, was removed from memory once the addxy function finished executing. However, the original variable `total` remains 0 when we print its value from main.
Now we subtract y from x using the subxy function. This time we pass variable `total` by reference (using the “address of” operator (&)) to the subxy function.
Note how we use the dereference operator “*” to get the value of total inside the function. This is necessary, because you want the value in variable `total`, not the address of variable `total` that was passed in.
Now we print the value of variable `total` from main again after the subxy function finishes. We get a value of 5, which matches the value of total printed from inside of subxy function. The reason is because by passing a variabletotal by reference we did not make a copy of the variable `total` instead we passed the address in memory of the same variable `total` used in the function main. In other words we only had one total variable during entire code execution time.
0 comments:
Post a Comment